· 10 min read
Enterprise Software Design Patterns Guide
a comprehensive guide to enterprise software design patterns.
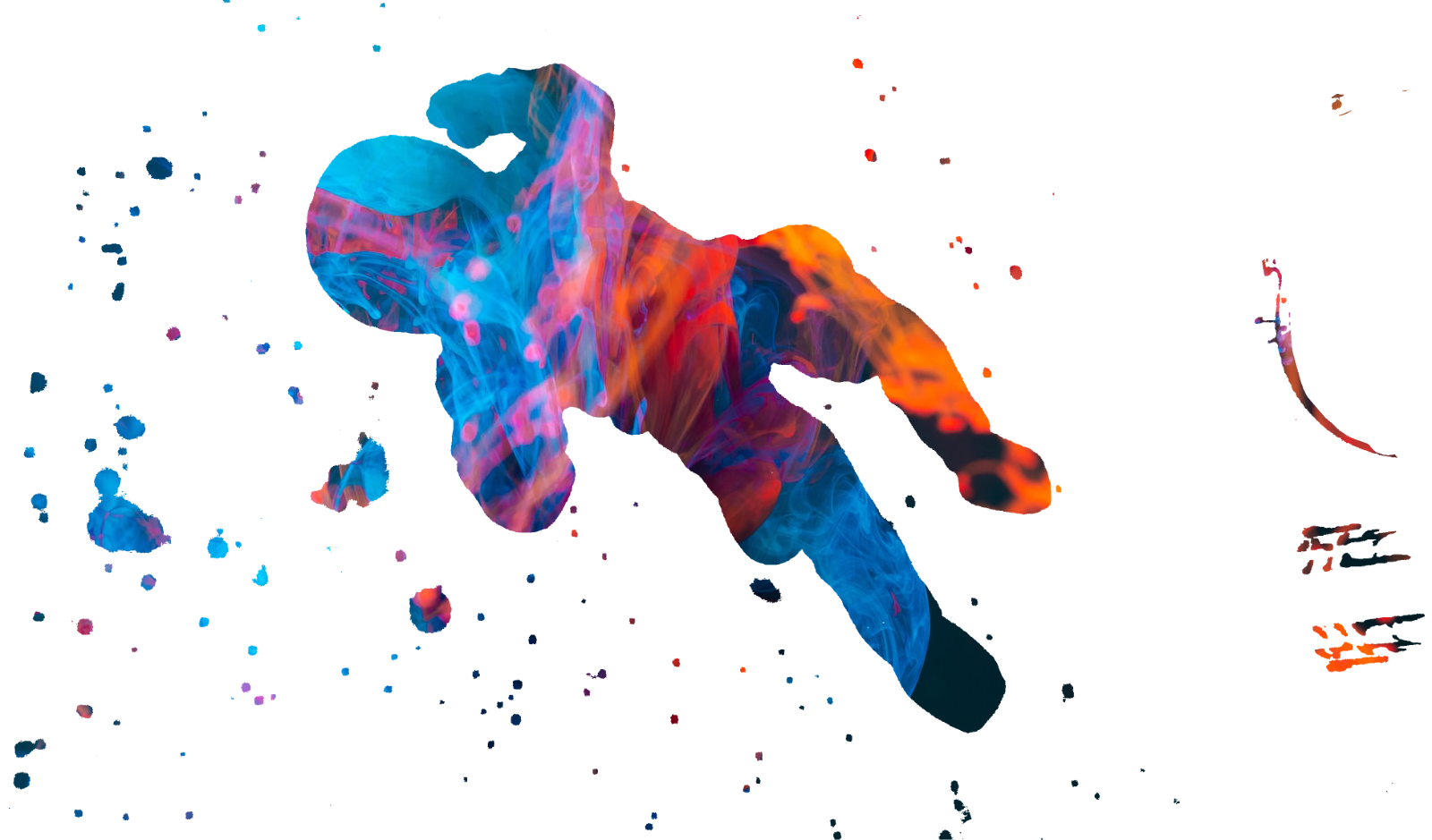
Enterprise Software Design Patterns Guide
Creational Patterns
Singleton Pattern
Purpose: Ensures a class has only one instance and provides a global point of access to it.
Use Cases:
- Database connection pools
- Configuration managers
- Logging services
Implementation Example:
public class DatabaseConnection {
private static DatabaseConnection instance;
private Connection connection;
private DatabaseConnection() {
// Private constructor to prevent instantiation
}
public static synchronized DatabaseConnection getInstance() {
if (instance == null) {
instance = new DatabaseConnection();
}
return instance;
}
}
Benefits:
- Controls access to shared resources
- Ensures single point of responsibility
- Reduces memory footprint
Drawbacks:
- Can make unit testing difficult
- May introduce global state
- Thread safety concerns
Factory Method Pattern
Purpose: Defines an interface for creating objects but lets subclasses decide which class to instantiate.
Use Cases:
- Document generators (PDF, Word, Excel)
- Payment processing systems
- UI component creation
Implementation Example:
public interface PaymentProcessor {
void processPayment(double amount);
}
public class PaymentProcessorFactory {
public PaymentProcessor createProcessor(String type) {
switch(type) {
case "CREDIT":
return new CreditCardProcessor();
case "DEBIT":
return new DebitCardProcessor();
case "CRYPTO":
return new CryptoProcessor();
default:
throw new IllegalArgumentException("Unknown payment type");
}
}
}
Abstract Factory Pattern
Purpose: Provides an interface for creating families of related objects without specifying their concrete classes.
Use Cases:
- Cross-platform UI components
- Database access layer for multiple databases
- Cloud service providers integration
Implementation Example:
public interface CloudServiceFactory {
Storage createStorage();
Compute createCompute();
Network createNetwork();
}
public class AWSServiceFactory implements CloudServiceFactory {
public Storage createStorage() {
return new S3Storage();
}
public Compute createCompute() {
return new EC2Compute();
}
public Network createNetwork() {
return new VPCNetwork();
}
}
Structural Patterns
Adapter Pattern
Purpose: Converts the interface of a class into another interface clients expect.
Use Cases:
- Legacy system integration
- Third-party library integration
- API compatibility layers
Implementation Example:
public interface ModernPaymentGateway {
void processPayment(Payment payment);
}
public class LegacyPaymentSystem {
public void makePayment(String amount, String currency) {
// Legacy payment logic
}
}
public class PaymentSystemAdapter implements ModernPaymentGateway {
private LegacyPaymentSystem legacySystem;
public void processPayment(Payment payment) {
String amount = payment.getAmount().toString();
String currency = payment.getCurrency();
legacySystem.makePayment(amount, currency);
}
}
Decorator Pattern
Purpose: Attaches additional responsibilities to objects dynamically.
Use Cases:
- Input/Output streams
- UI component enhancement
- Service layer functionality extension
Implementation Example:
public interface DataSource {
void writeData(String data);
String readData();
}
public class EncryptionDecorator implements DataSource {
private DataSource wrappee;
public void writeData(String data) {
String encrypted = encrypt(data);
wrappee.writeData(encrypted);
}
public String readData() {
String encrypted = wrappee.readData();
return decrypt(encrypted);
}
}
Behavioral Patterns
Observer Pattern
Purpose: Defines a one-to-many dependency between objects where all dependents are notified of state changes.
Use Cases:
- Event handling systems
- Message queuing systems
- Real-time data monitoring
Implementation Example:
public interface Observer {
void update(String event);
}
public class EventManager {
private List<Observer> observers = new ArrayList<>();
public void subscribe(Observer observer) {
observers.add(observer);
}
public void notify(String event) {
for(Observer observer : observers) {
observer.update(event);
}
}
}
Strategy Pattern
Purpose: Defines a family of algorithms and makes them interchangeable.
Use Cases:
- Sorting algorithms
- Payment processing methods
- Data compression techniques
Implementation Example:
public interface ValidationStrategy {
boolean validate(String data);
}
public class DataValidator {
private ValidationStrategy strategy;
public void setStrategy(ValidationStrategy strategy) {
this.strategy = strategy;
}
public boolean validateData(String data) {
return strategy.validate(data);
}
}
Integration Patterns
Gateway Pattern
Purpose: Provides a unified interface to a set of interfaces in a subsystem.
Use Cases:
- External API integration
- Payment gateway implementations
- Service aggregation
Implementation Example:
public class PaymentGateway {
private Map<String, PaymentProvider> providers;
public PaymentResponse processPayment(PaymentRequest request) {
PaymentProvider provider = providers.get(request.getProvider());
return provider.process(request);
}
}
Circuit Breaker Pattern
Purpose: Prevents cascading failures in distributed systems.
Use Cases:
- Microservice communication
- External API calls
- Database operations
Implementation Example:
public class CircuitBreaker {
private int failureThreshold;
private int failureCount;
private State state;
public Response execute(Function<Request, Response> action) {
if (state == State.OPEN) {
throw new CircuitBreakerOpenException();
}
try {
Response response = action.apply(request);
reset();
return response;
} catch (Exception e) {
handleFailure();
throw e;
}
}
}
Distributed System Patterns
Saga Pattern
Purpose: Manages data consistency across microservices in distributed transactions.
Use Cases:
- E-commerce order processing
- Travel booking systems
- Financial transactions
Implementation Example:
public class OrderSaga {
private final OrderService orderService;
private final PaymentService paymentService;
private final InventoryService inventoryService;
public void createOrder(Order order) {
try {
// Start saga
orderService.createOrder(order);
paymentService.processPayment(order);
inventoryService.updateInventory(order);
orderService.completeOrder(order);
} catch (Exception e) {
// Compensating transactions
compensate(order);
}
}
private void compensate(Order order) {
// Reverse completed steps
}
}
Event Sourcing Pattern
Purpose: Stores the state of business entities as a sequence of immutable events.
Use Cases:
- Audit logging
- Regulatory compliance
- Complex domain models with history tracking
Implementation Example:
public class AccountEventStore {
private final EventStore eventStore;
public void saveEvent(AccountEvent event) {
eventStore.append(event);
}
public Account reconstructAccount(String accountId) {
List<Event> events = eventStore.getEvents(accountId);
Account account = new Account();
for(Event event : events) {
account.apply(event);
}
return account;
}
}
Bulkhead Pattern
Purpose: Isolates elements of an application into pools so that if one fails, the others will continue to function.
Use Cases:
- Resource pool management
- Service isolation
- Fault containment
Implementation Example:
public class BulkheadExecutor {
private final Map<String, Semaphore> semaphores = new ConcurrentHashMap<>();
public <T> CompletableFuture<T> execute(String poolKey, Supplier<T> supplier) {
Semaphore semaphore = semaphores.get(poolKey);
return CompletableFuture.supplyAsync(() -> {
try {
semaphore.acquire();
return supplier.get();
} finally {
semaphore.release();
}
});
}
}
Sidecar Pattern
Purpose: Deploys components of an application as a separate process or container to provide isolation and encapsulation.
Use Cases:
- Service mesh implementations
- Logging and monitoring
- Security features
Implementation Example:
public class ServiceProxy {
private final Service mainService;
private final MetricsCollector metricsCollector;
private final SecurityHandler securityHandler;
public Response processRequest(Request request) {
// Pre-processing
securityHandler.validateRequest(request);
metricsCollector.recordRequest(request);
// Main service call
Response response = mainService.handle(request);
// Post-processing
metricsCollector.recordResponse(response);
return response;
}
}
Backend for Frontend (BFF) Pattern
Purpose: Creates separate backend services for specific frontend applications.
Use Cases:
- Mobile applications
- Web applications
- IoT devices
Implementation Example:
public class MobileBackendService {
private final UserService userService;
private final ContentService contentService;
public MobileUserResponse getUserProfile(String userId) {
User user = userService.getUser(userId);
Content content = contentService.getOptimizedContent(userId);
return new MobileUserResponse(user, content);
}
}
API Gateway Pattern
Purpose: Provides a single entry point for certain groups of microservices.
Use Cases:
- Request routing
- API composition
- Protocol translation
Implementation Example:
public class ApiGateway {
private final ServiceRegistry registry;
private final LoadBalancer loadBalancer;
public Response routeRequest(Request request) {
Service service = registry.getService(request.getPath());
Instance instance = loadBalancer.getInstance(service);
return instance.processRequest(
transformRequest(request)
);
}
}
Sharding Pattern
Purpose: Divides a data store into a set of horizontal partitions or shards.
Use Cases:
- Large-scale databases
- High-throughput systems
- Geographically distributed applications
Implementation Example:
public class ShardingRouter {
private final Map<Integer, DataSource> shards;
public DataSource getShardForKey(String key) {
int shardIndex = calculateShardIndex(key);
return shards.get(shardIndex);
}
private int calculateShardIndex(String key) {
return Math.abs(key.hashCode() % shards.size());
}
}
Service Discovery Pattern
Purpose: Enables automatic detection of services and their locations in a distributed system.
Use Cases:
- Microservices architecture
- Cloud-native applications
- Dynamic service routing
Implementation Example:
public class ServiceRegistry {
private Map<String, List<ServiceInstance>> services = new ConcurrentHashMap<>();
public void register(String serviceName, ServiceInstance instance) {
services.computeIfAbsent(serviceName, k -> new CopyOnWriteArrayList<>())
.add(instance);
}
public List<ServiceInstance> discover(String serviceName) {
return services.getOrDefault(serviceName, Collections.emptyList());
}
public void heartbeat(String serviceName, String instanceId) {
// Update last seen timestamp
}
}
Rate Limiting Pattern
Purpose: Controls the rate of requests to protect services from overload.
Use Cases:
- API management
- DDoS protection
- Resource consumption control
Implementation Example:
public class TokenBucketRateLimiter {
private final long capacity;
private final double refillRate;
private double tokens;
private long lastRefillTime;
public synchronized boolean tryAcquire() {
refill();
if (tokens >= 1) {
tokens--;
return true;
}
return false;
}
private void refill() {
long now = System.nanoTime();
double tokensToAdd = (now - lastRefillTime) * refillRate;
tokens = Math.min(capacity, tokens + tokensToAdd);
lastRefillTime = now;
}
}
Backpressure Pattern
Purpose: Manages flow control when a producer creates data faster than consumers can process it.
Use Cases:
- Stream processing
- Message queuing systems
- Real-time data processing
Implementation Example:
public class BackpressureBuffer<T> {
private final BlockingQueue<T> queue;
private final int highWaterMark;
private final int lowWaterMark;
public void produce(T item) throws InterruptedException {
while (queue.size() >= highWaterMark) {
// Wait for consumer to catch up
Thread.sleep(100);
}
queue.put(item);
}
public T consume() throws InterruptedException {
T item = queue.take();
if (queue.size() <= lowWaterMark) {
// Signal producer to resume
notifyProducers();
}
return item;
}
}
Circuit Breaker with Advanced Features
Purpose: Prevents system failures by detecting and isolating failing services with sophisticated recovery mechanisms.
Use Cases:
- Microservices communication
- External API calls
- Fault tolerance
Implementation Example:
public class AdvancedCircuitBreaker {
private final String name;
private final int failureThreshold;
private final long resetTimeout;
private final AtomicInteger failureCount;
private final AtomicReference<CircuitState> state;
private volatile long lastFailureTime;
public <T> T execute(Supplier<T> action, Supplier<T> fallback) {
if (isOpen()) {
if (shouldAttemptReset()) {
// Try half-open state
return executeWithHalfOpen(action, fallback);
}
return fallback.get();
}
try {
T result = action.get();
reset();
return result;
} catch (Exception e) {
handleFailure();
return fallback.get();
}
}
private <T> T executeWithHalfOpen(Supplier<T> action, Supplier<T> fallback) {
if (state.compareAndSet(CircuitState.OPEN, CircuitState.HALF_OPEN)) {
try {
T result = action.get();
reset();
return result;
} catch (Exception e) {
moveToOpen();
return fallback.get();
}
}
return fallback.get();
}
}
Concurrent Processing Patterns
Producer-Consumer Pattern
Purpose: Separates the production and consumption of data into separate threads or processes.
Implementation Example:
public class ProducerConsumerQueue<T> {
private final BlockingQueue<T> queue;
private final int numConsumers;
private final ExecutorService executorService;
public void startProcessing(Producer<T> producer, Consumer<T> consumer) {
// Start producer
executorService.submit(() -> {
while (true) {
T item = producer.produce();
queue.put(item);
}
});
// Start consumers
for (int i = 0; i < numConsumers; i++) {
executorService.submit(() -> {
while (true) {
T item = queue.take();
consumer.consume(item);
}
});
}
}
}
Work Stealing Pattern
Purpose: Enables efficient load balancing in parallel processing scenarios.
Implementation Example:
public class WorkStealingPool {
private final Deque<Runnable>[] queues;
private final Random random = new Random();
public void submit(Runnable task) {
int workerId = Thread.currentThread().getId();
queues[workerId].addLast(task);
}
public Runnable steal() {
int victimId = random.nextInt(queues.length);
return queues[victimId].pollLast();
}
}
Fork-Join Pattern
Purpose: Efficiently processes recursive algorithms in parallel.
Implementation Example:
public class ParallelArrayProcessor extends RecursiveTask<Integer> {
private final int[] array;
private final int start;
private final int end;
private final int threshold;
@Override
protected Integer compute() {
if (end - start <= threshold) {
return processSequentially();
}
int mid = (start + end) / 2;
ParallelArrayProcessor leftTask = new ParallelArrayProcessor(array, start, mid);
ParallelArrayProcessor rightTask = new ParallelArrayProcessor(array, mid, end);
leftTask.fork();
int rightResult = rightTask.compute();
int leftResult = leftTask.join();
return leftResult + rightResult;
}
}
Parallel Processing Patterns
MapReduce Pattern
Purpose: Processes large datasets in parallel by dividing work into map and reduce phases.
Implementation Example:
public class MapReduceFramework {
private final ExecutorService mapPool;
private final ExecutorService reducePool;
public <T, R> List<R> process(List<T> input,
Function<T, R> mapper,
BinaryOperator<R> reducer) {
// Map phase
List<Future<R>> mappedResults = input.stream()
.map(item -> mapPool.submit(() -> mapper.apply(item)))
.collect(Collectors.toList());
// Reduce phase
return reducePool.submit(() ->
mappedResults.stream()
.map(this::getFutureResult)
.reduce(reducer)
.orElseThrow()
).get();
}
}
Scatter-Gather Pattern
Purpose: Distributes parallel processing tasks and aggregates results.
Implementation Example:
public class ScatterGatherProcessor<T, R> {
private final ExecutorService executorService;
private final int numWorkers;
public R process(List<T> items, Function<T, R> processor,
Function<List<R>, R> aggregator) {
// Scatter
List<Future<R>> futures = new ArrayList<>();
for (List<T> batch : partition(items, numWorkers)) {
futures.add(executorService.submit(() ->
processor.apply(batch.get(0))));
}
// Gather
List<R> results = futures.stream()
.map(this::getFutureResult)
.collect(Collectors.toList());
return aggregator.apply(results);
}
}
Architectural Patterns
Repository Pattern
Purpose: Mediates between the domain and data mapping layers.
Use Cases:
- Database access abstraction
- Data persistence operations
- Domain object management
Implementation Example:
public interface UserRepository {
User findById(Long id);
void save(User user);
void delete(User user);
List<User> findAll();
}
public class JpaUserRepository implements UserRepository {
@PersistenceContext
private EntityManager em;
public User findById(Long id) {
return em.find(User.class, id);
}
// Other implementation methods
}
CQRS Pattern
Purpose: Separates read and write operations for a data store.
Use Cases:
- High-performance applications
- Complex domain models
- Event-sourced systems
Implementation Example:
public class OrderCommandService {
public void createOrder(CreateOrderCommand command) {
// Handle write operations
}
}
public class OrderQueryService {
public OrderDTO getOrder(Long orderId) {
// Handle read operations
}
}
Best Practices
Pattern Selection:
- Choose patterns based on specific requirements
- Consider maintenance implications
- Avoid over-engineering
Implementation Guidelines:
- Keep implementations simple
- Document pattern usage
- Consider performance implications
Common Anti-patterns to Avoid:
- God Object
- Golden Hammer
- Spaghetti Code
Conclusion
Design patterns are essential tools in enterprise software development, but they should be used judiciously. The key is to understand:
- When to apply each pattern
- The tradeoffs involved
- How patterns interact with each other
Remember that patterns should solve problems, not create them. Always consider the specific context and requirements of your application when choosing and implementing design patterns.