· 4 min read
Java enum
Java enum is a powerful feature that goes beyond just defining a fixed set of constants.
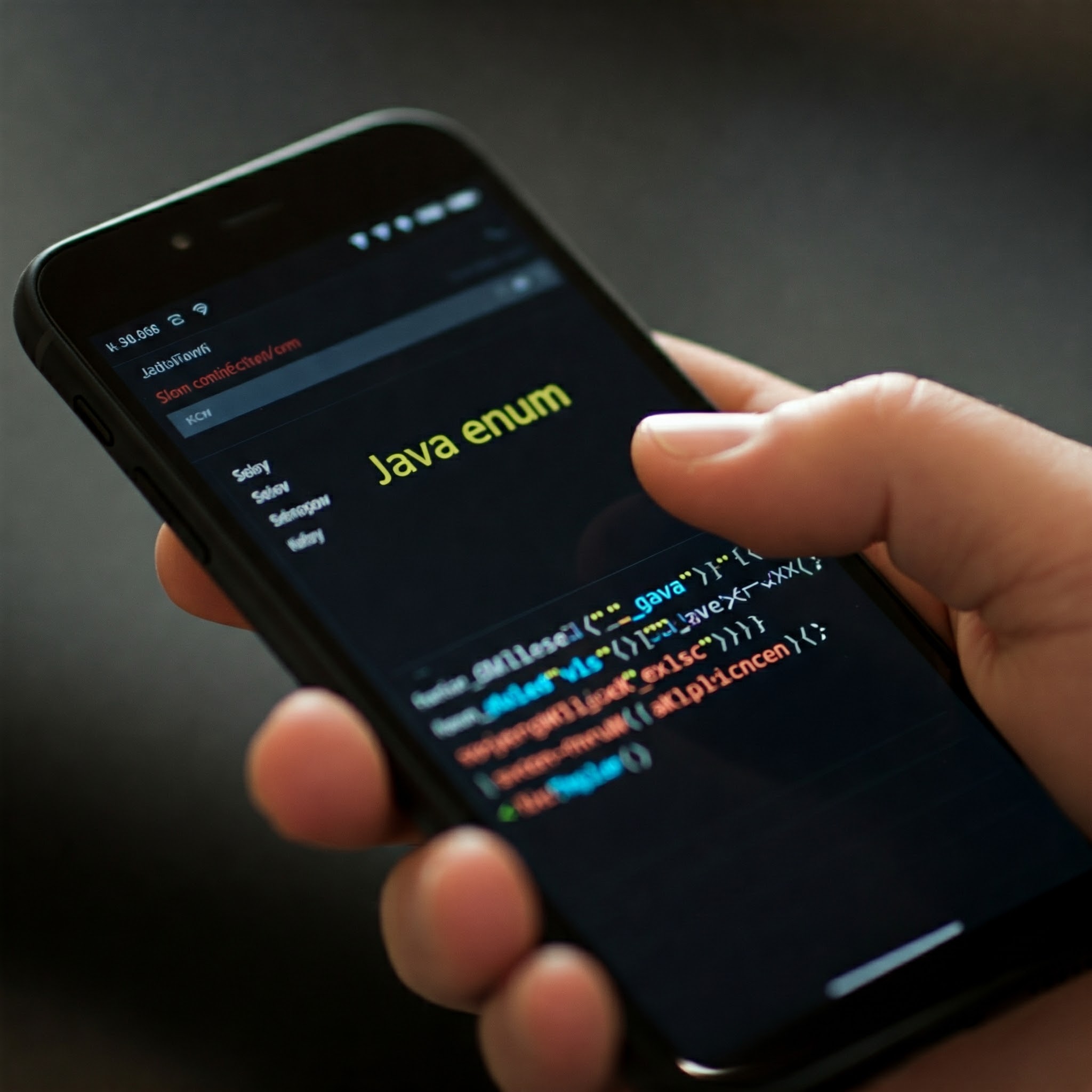
Java enum
is a powerful feature that goes beyond just defining a fixed set of constants. Below are some advanced features of Java enum
, explained with examples:
1. Adding Fields, Constructors, and Methods
Enums can have fields, constructors, and methods. Each constant can have its own properties.
enum Direction {
NORTH("Up"), SOUTH("Down"), EAST("Right"), WEST("Left");
private final String description;
// Constructor
Direction(String description) {
this.description = description;
}
// Getter method
public String getDescription() {
return description;
}
}
public class EnumDemo {
public static void main(String[] args) {
for (Direction dir : Direction.values()) {
System.out.println(dir + ": " + dir.getDescription());
}
}
}
Output:
NORTH: Up
SOUTH: Down
EAST: Right
WEST: Left
2. Overriding Methods for Each Constant
Enums can override methods differently for each constant.
enum Operation {
ADD {
@Override
public int apply(int x, int y) {
return x + y;
}
},
SUBTRACT {
@Override
public int apply(int x, int y) {
return x - y;
}
},
MULTIPLY {
@Override
public int apply(int x, int y) {
return x * y;
}
},
DIVIDE {
@Override
public int apply(int x, int y) {
return x / y;
}
};
// Abstract method to be implemented by each constant
public abstract int apply(int x, int y);
}
public class EnumDemo {
public static void main(String[] args) {
int x = 10, y = 5;
for (Operation op : Operation.values()) {
System.out.println(op + ": " + op.apply(x, y));
}
}
}
Output:
ADD: 15
SUBTRACT: 5
MULTIPLY: 50
DIVIDE: 2
3. Using Enum with Switch Statement
Enums integrate seamlessly with switch
statements.
enum Day {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY
}
public class EnumSwitchDemo {
public static void main(String[] args) {
Day today = Day.FRIDAY;
switch (today) {
case MONDAY:
case TUESDAY:
case WEDNESDAY:
case THURSDAY:
case FRIDAY:
System.out.println("It's a weekday.");
break;
case SATURDAY:
case SUNDAY:
System.out.println("It's the weekend!");
break;
}
}
}
Output:
It's a weekday.
4. Implementing Interfaces
Enums can implement interfaces, making them even more versatile.
interface Printable {
void print();
}
enum Color implements Printable {
RED {
@Override
public void print() {
System.out.println("Color is Red");
}
},
GREEN {
@Override
public void print() {
System.out.println("Color is Green");
}
},
BLUE {
@Override
public void print() {
System.out.println("Color is Blue");
}
};
}
public class EnumInterfaceDemo {
public static void main(String[] args) {
for (Color color : Color.values()) {
color.print();
}
}
}
Output:
Color is Red
Color is Green
Color is Blue
5. Using Enum Constants with Behavior and Properties
Enums can have constants that behave differently based on their properties.
enum Planet {
MERCURY(3.3011e23, 2.4397e6),
VENUS(4.8675e24, 6.0518e6),
EARTH(5.97237e24, 6.371e6),
MARS(6.4171e23, 3.3895e6);
private final double mass; // in kilograms
private final double radius; // in meters
Planet(double mass, double radius) {
this.mass = mass;
this.radius = radius;
}
public double surfaceGravity() {
final double G = 6.67430e-11; // gravitational constant
return G * mass / (radius * radius);
}
}
public class EnumPlanetDemo {
public static void main(String[] args) {
for (Planet planet : Planet.values()) {
System.out.printf("Surface gravity on %s is %.2f m/s²%n",
planet, planet.surfaceGravity());
}
}
}
Output (sample values):
Surface gravity on MERCURY is 3.70 m/s²
Surface gravity on VENUS is 8.87 m/s²
Surface gravity on EARTH is 9.80 m/s²
Surface gravity on MARS is 3.71 m/s²
6. EnumSet and EnumMap
Enums work seamlessly with collections like EnumSet
and EnumMap
.
EnumSet Example:
import java.util.EnumSet;
enum Size { SMALL, MEDIUM, LARGE, EXTRALARGE }
public class EnumSetDemo {
public static void main(String[] args) {
EnumSet<Size> sizes = EnumSet.of(Size.SMALL, Size.LARGE);
for (Size size : sizes) {
System.out.println(size);
}
}
}
EnumMap Example:
import java.util.EnumMap;
enum Level { LOW, MEDIUM, HIGH }
public class EnumMapDemo {
public static void main(String[] args) {
EnumMap<Level, String> levelDescriptions = new EnumMap<>(Level.class);
levelDescriptions.put(Level.LOW, "Low level");
levelDescriptions.put(Level.MEDIUM, "Medium level");
levelDescriptions.put(Level.HIGH, "High level");
for (Level level : levelDescriptions.keySet()) {
System.out.println(level + ": " + levelDescriptions.get(level));
}
}
}
Output:
LOW: Low level
MEDIUM: Medium level
HIGH: High level
These advanced features make enum
a highly versatile and powerful feature in Java, enabling developers to create robust and readable code.